2021/01/11 - [React] - [React] ReactDOM 을 사용하여 팝업창 띄우기
[React] ReactDOM 을 사용하여 팝업창 띄우기
오늘은 react-dom 을 사용하여 팝업창 생성을 해보도록 하자. react 프로젝트가 생성될 때 하나의 돔이 생성되고 그 위에 view 가 그려진다. 따라서 팝업창을 생성하기 위해선 이미 펼져쳐 있는 dom 위
ddeck.tistory.com
이전에 react-dom 을 이용하여 팝업창 띄우기를 공부했다.
이 것을 응용하여 loading 중 아이콘도 구현할 수 있다.
동일하게 this.state값을 이용하여 로딩중 아이콘이 있는 팝업창을 나타낼 것이다.
import React, {Component} from 'react'; import PopupDom from './PopupDom'; import Loading from './Loading'; import './Style.css' class Main extends Component { constructor(props){ super(props); this.state = { isOpenPopup: false, } this.openPopup = this.openPopup.bind(this); this.closePopup = this.closePopup.bind(this); } openPopup(){ this.setState({ isOpenPopup: true, }) } closePopup(){ this.setState({ isOpenPopup: false, }) } render(){ return( <div> <h2>Open Loading</h2> <div> <button type="button" id="popupDom" onClick={this.openPopup} > Click </button> {this.state.isOpenPopup && <PopupDom> <Loading onClose={this.closePopup}/> </PopupDom> } </div> </div> ); } } export default Main;
팝업창을 만들어줄 새로운 dom 도 생성한다.
import ReactDom from 'react-dom'; const PopupDom = ({ children }) => { const el = document.getElementById('popupDom'); return ReactDom.createPortal(children, el); }; export default PopupDom;
아래와 같이 loading 중 아이콘을 나타내주는 페이지를 작성한다.
나는 아이콘을 생성하지 않고 웹서핑으로 얻은 아이콘 이미지를 css-animation으로 돌려줄 것이다.
import React, {Component} from 'react'; class PopupContent extends Component { render(){ return( <div className="dimmed_layer_wrapper"> <div className="full_layer"> <div className="common_alert_loading"> <div>Loading....</div> <div className="loading"></div> <div> <button type="button" onClick={this.props.onClose}>close</button> </div> </div> </div> </div> ); } } export default PopupContent;
실무에서는 해당 팝업창에 버튼과 loading... 텍스트는 사용하지 않고 이미지만 넣을 것이다.
이번 글에서는 팝업창 구현 확인을 위하여 같이 적어 주었다.
실제 아이콘이 들어갈 곳은 이 곳이다.
<div className="loading"></div>
이제 모든 css 작업만 해주면 완료 된다.
애니메이션 구현을 위해 아래와 같이 작성한다.
@keyframes rotator { 0% { -webkit-transform: rotate(-45deg) translateZ(0); transform: rotate(-45deg) translateZ(0); } 100% { -webkit-transform: rotate(315deg) translateZ(0); transform: rotate(315deg) translateZ(0); } }
위와 같이 애니메이션 구현을 위한 코드에 rotator 라고 이름을 주었다.
이 코드를 이용하여 애니메이션 효과를 줄 수 있다.
.common_alert_loading { z-index:2; position:relative; display:inline-block; vertical-align:middle; width:calc(100%-56px); min-height: 200px; max-height:100%; min-width: 200px; white-space:normal; word-break:break-word; text-align:left; padding:30px; margin:auto; box-sizing:border-box; font-size: 15px; } .loading { background-size: 100px 100px; background-image: url('./loading.png'); width: 100px; height: 100px; margin: 20px; animation-name: rotator; animation-iteration-count: infinite; animation-duration: 3s; }
위의 코드에서 animation-name 으로 우리가 애니메이션 설정했던 rotator 로 지정해 주었다.
animation-duration 은 한번 구현될 때 걸리는 속도를 의미하고, animation-iteration-count 로 애니메이션 횟수를 지정해 줄 수 있다. 나는 infinite 를 사용하여 계속 돌려주었다.
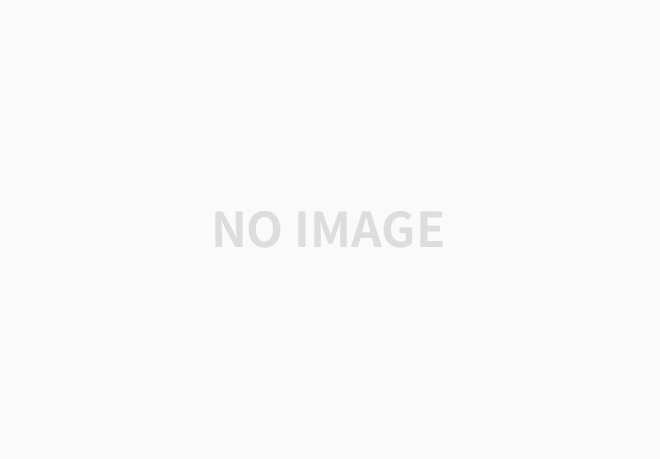
'React' 카테고리의 다른 글
[React] 절대경로를 사용하여 css 적용하기 - 떽떽대는 개발공부 (0) | 2021.01.15 |
---|---|
[React] React webpack 을 이용하여 개발 환경 구성하기 - 떽떽대는 개발공부 (0) | 2021.01.14 |
[React] ReactDOM 을 사용하여 팝업창 띄우기 - 떽떽대는 개발공부 (0) | 2021.01.11 |
[React] a태그 onClick 이벤트 render 에러 해결 - 떽떽대는 개발공부 (0) | 2021.01.07 |
[React] react-toasts 의 style 변경하기 - 떽떽대는 개발공부 (0) | 2021.01.06 |
댓글